invert binary tree solution
Class Solution public. Define a queue Q.
Add root node to queue Q.
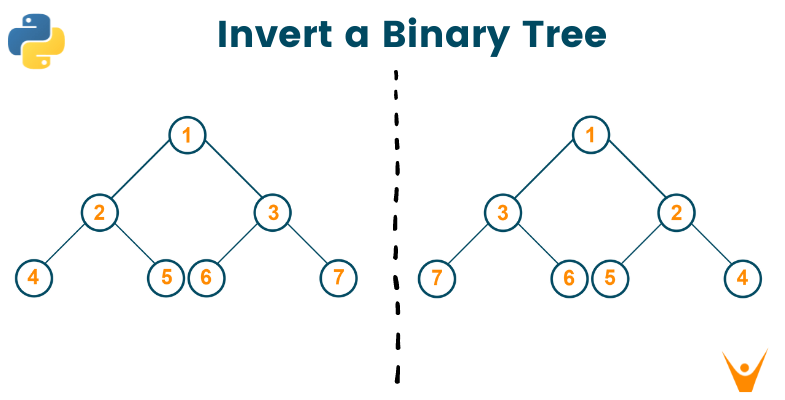
. Initialize a queue to store tree nodes queue Return None if input tree is None if tree None. So if the tree is like below. Def invertBinaryTree tree.
Swap left and right child of node N. Return None Add root node into queue queueappendtree Loop while the queue has nodes while len queue 0. The number of nodes in the tree is in the range 0 100-100.
Related
TreeNode invertTreeTreeNode root ifroot NULL return NULL. We traverse through all n nodes using recursion for On time complexity and we can have up to logn recursive calls on the stack at once where logn is the depth of the tree for Ologn space complexity. Below are the three approaches to solve this problem.
Traverse the tree in pre-order or post-order way. We can ask ourselves which tree traversal would be best to invert the binary tree. When both trees have been traversed swap left and right child subtrees.
Suppose we have a binary tree. Merge Two Binary Trees by doing Node Sum Recursive and Iterative Vertical Sum in a given Binary Tree Set 1. Traverses the right subtree.
And in each iteration swap its left and child and then invert its left and right subtree by calling them recursively. The inverted binary tree. Given a binary tree invert it and return the new value.
Hint 2 Once the first swap mentioned in Hint 1 is done you must invert the root nodes left child node and its right child node. Curr_node stackpop if curr_nodeleft None or curr_noderight None. Invert Tree - Solution.
TreeNode r invertTreeroot-right. Given the root of a binary tree invert the tree and return its root. Let n be the number of nodes in the binary tree.
Invert Binary Tree using Level Order Traversal Java Code. Root 4271369 Output. Our task is to create an inverted binary tree.
Invert Binary Tree LeetCode Solution In this question Given a root of any binary tree the. A binary tree that needs to be inverted. Adding that to our code looks like this.
You may invert it in-place. An inverted Binary Tree is simply a Binary Tree whose left and right children are swapped. Inverting this root node simply consists of swapping its left and right child nodes which can be done the same way as swapping two variables.
Selfval x selfleft None selfright None class Solutionobject. An inversion also known as a mirror of a Binary Tree T is simply a Binary Tree M T in which the left and right children of all non-leaf nodes have been flipped around. Public TreeNode invertTree TreeNode root helper root.
Public TreeNode invertTree TreeNode root helper root. Public void helper TreeNode n if nnull return. Complexity of Final Solution.
Pop node N from queue Q from left side. Root Output. TreeNode if root None.
Traverses the left subtree. Function to invert given binary Tree using preorder traversal void invertBinaryTreeTreeNode root base case. Converting recursive approach to iterative by using stack.
Def __init__self x. Stack stackappendroot while stack. Recursively solve left subtree and right subtree.
Invert the binary tree recursively. Find largest subtree sum in a tree. Invert binary tree video tutorial.
Vertical Sum in Binary Tree Set 2 Space Optimized Find root of the tree where children id sum for every node is given. If the root is null then return. The inverted tree will be like.
Root 4271369 Output. You can invert a binary tree using recursive and iterative approaches. In level order traversal We traverse a given binary tree level by level.
In this section I am going to discuss how we can solve this problem using the level order traversal of a binary tree. Grab node off the queue treeNode queuepop Swap the children nodes if treeNode None. If root current node is NULL inverting is done.
Root Output. We can easily convert the above recursive solution into an iterative one using a queue or stack to store tree nodes. The problem is pretty simple invert a tree or in other words create a mirror image of the tree.
If tree is empty if root Null return swap left subtree with right subtree swap rootleft rootright invert left subtree invertBinaryTree rootleft invert right subtree invertBinaryTree rootright. Public void helper TreeNode n if n null return. Var invertTree functionroot const reverseNode node if node null return null reverseNode nodeleft.
To visualize the solution and step through the below code click Visualize the. Root 213 Output. Hint 1 Start by inverting the root node of the Binary Tree.
This is our final solution. And To invert them interchange their left and right children of a node. TreeNode l invertTreeroot-left.
Using Iterative preorder traversal. Definition for a binary tree node. Inverting an empty tree does nothing.
Replace each node in binary tree with the sum of its inorder predecessor and successor. The answer is a straightforward recursive procedure. To understand the problem a basic knowledge of binary tree is required.
Swap the left and right pointers. 1 tree. The implementation of the tree is given and is nothing different from the usual containing left and right child for each node.
Java Solution 1 - Recursive. Swapping the left and right child of every node in subtree recursively. TreeNode t n.
Given the root of a binary tree invert the tree and return its root. To invert a binary tree switch the left subtree and the right subtree and invert them both. Subtree inversion for the right-subtree.
Invert Binary Tree LeetCode Solution Problem Statement. Subtree inversion for the left subtree. Steps to invert a Binary Tree iteratively using Queue.
While queue Q is not empty. This is demonstrated below in C Java and Python. The number of nodes in the tree is in the range 0 100-100.
After that we obviously have to actually reverse the node which means we will need to swap the left and right values. Swap the left and right subtrees. The code is almost similar to the level order traversal of a binary tree.
The program requires Oh extra space for the call stack where h is the height of the tree. Root 213 Output. To solve this we will use a recursive approach.
Python Inverting Binary Tree Recursive Stack Overflow
Invert A Binary Tree Python Code With Example Favtutor
Coding Short Inverting A Binary Tree In Python By Theodore Yoong Medium
Leetcode Invert Binary Tree Solution Explained Java Youtube
Invert Binary Tree Iterative Recursive Approach
Invert Alternate Levels Of A Perfect Binary Tree Techie Delight
Invert A Binary Tree Python Code With Example Favtutor
Algodaily Invert A Binary Tree Description
Invert A Binary Tree Python Code With Example Favtutor
Invert Binary Tree Leetcode 226 Youtube
Invert Binary Tree Iterative And Recursive Solution Techie Delight
A Visual Guide To How To Actually Invert A Binary Tree Dev Community
How To Invert A Binary Tree In C C Algorithms Blockchain And Cloud
Algodaily Invert A Binary Tree Description
Invert A Binary Tree Python Code With Example Favtutor
Depth First Search Invert Binary Tree In C Stack Overflow
Invert A Binary Tree Recursive And Iterative Solutions Learnersbucket
How To Invert Binary Tree Java The Coding Shala